Shadcn UI: A Comprehensive Guide of Opensouce UI
Introduction
In the ever-evolving landscape of web development, creating visually appealing and functionally robust user interfaces remains a paramount challenge. Enter Shadcn UI, a game-changing toolkit that has been making waves in the developer community. This innovative UI library has emerged as a powerful solution for crafting beautiful, accessible, and highly customizable user interfaces with remarkable ease.
Shadcn UI represents a paradigm shift in how developers approach UI design and implementation. By offering a collection of meticulously crafted components, it bridges the gap between design and development, enabling creators to build sophisticated interfaces without sacrificing flexibility or performance.
As we delve deeper into the world of modern web development, the importance of tools like Shadcn UI cannot be overstated. In an era where user experience reigns supreme, the ability to rapidly prototype and deploy polished interfaces gives developers a significant edge. Shadcn UI not only accelerates the development process but also ensures consistency and accessibility across projects, addressing some of the most pressing concerns in contemporary web design.
This comprehensive guide aims to explore the multifaceted aspects of Shadcn UI, from its core concepts and components to advanced customization techniques and real-world applications. Whether you're a seasoned developer looking to streamline your workflow or a newcomer eager to elevate your UI game, this journey through Shadcn UI promises valuable insights and practical knowledge to enhance your web development toolkit.
What is Shadcn UI?
Shadcn UI is not just another UI library; it's a revolutionary approach to building user interfaces for web applications. At its core, Shadcn UI is a collection of re-usable components that are designed to be accessible, customizable, and easy to integrate into React projects.
Definition and Core Concept
Shadcn UI, created by developer and designer shadcn, is an open-source UI component library that provides a set of high-quality, customizable React components. Unlike traditional component libraries, Shadcn UI adopts a unique "copy and paste" approach. This means that instead of installing the entire library as a dependency, developers can selectively copy the components they need directly into their projects.
The philosophy behind Shadcn UI is to offer maximum flexibility and control to developers. By allowing direct access to the component source code, it empowers developers to customize and extend the components to fit their specific needs without being constrained by the limitations of a pre-packaged library.
Key Features and Benefits
-
Accessibility: All components are built with accessibility in mind, ensuring that your applications are usable by a wide range of users, including those with disabilities.
-
Customization: The copy-paste approach allows for deep customization. Developers can easily modify styles, behaviors, and even the core functionality of components.
-
TypeScript Support: Written in TypeScript, Shadcn UI provides excellent type safety and autocompletion, enhancing the developer experience.
-
Modern Design: The components follow modern design principles, offering a clean and professional look out of the box.
-
Performance: By allowing developers to include only the components they need, Shadcn UI helps keep application bundle sizes small and performance optimized.
-
Flexibility: It's not opinionated about styling solutions. While it uses Tailwind CSS by default, developers can adapt the components to work with other CSS frameworks or custom styling solutions.
-
Community-Driven: Being open-source, Shadcn UI benefits from community contributions, continuously evolving and improving.
-
Documentation: Comprehensive documentation and examples make it easy for developers to get started and make the most of the library.
-
Theming: Built-in dark mode support and easy theming capabilities allow for quick adaptation to various design requirements.
-
Framework Agnostic: While primarily designed for React, the concepts and styles can be adapted to other frameworks, making it a versatile choice for various project types.
Getting Started with Shadcn UI
Embarking on your journey with Shadcn UI is a straightforward process, but it's slightly different from traditional component libraries. Let's walk through the steps to get Shadcn UI up and running in your project.
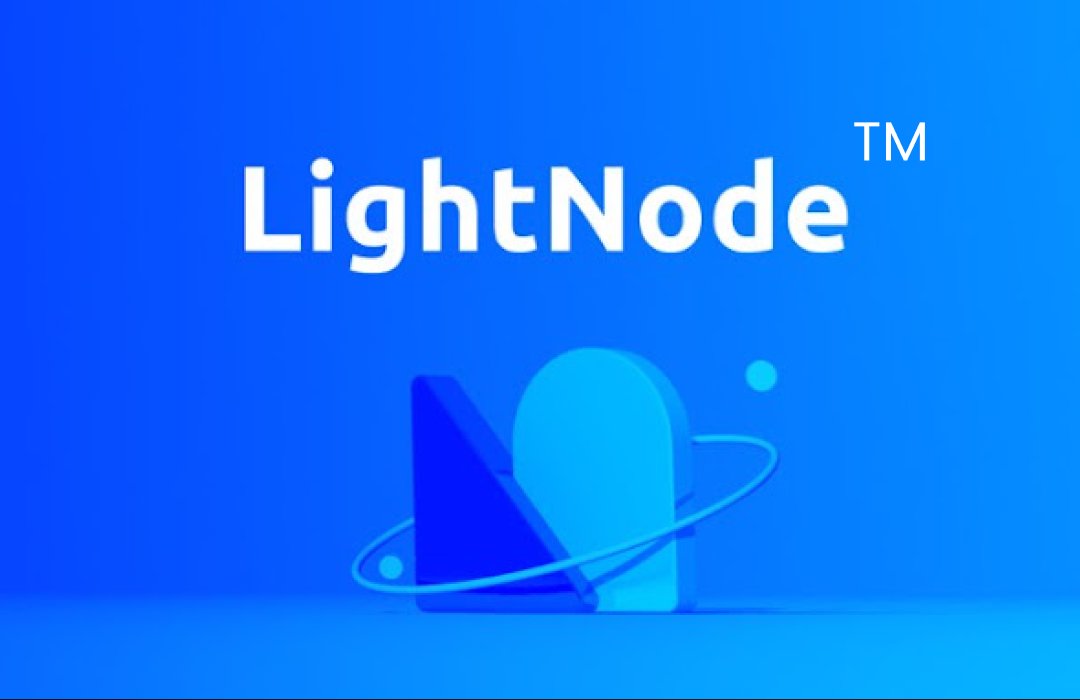
Buy Linux VPS by Hourly
Best Linux VPS with different Linux distributions.
Installation Process
Unlike conventional npm packages, Shadcn UI doesn't require a standard installation. Instead, you'll be using a CLI tool to add components to your project. Here's how to get started:
-
Create a New Project (if you don't have one already): You can use your preferred React framework. For example, with Next.js:
npx create-next-app@latest my-app cd my-app
-
Install Dependencies: Shadcn UI requires a few peer dependencies. Install them using your package manager:
npm install tailwindcss autoprefixer postcss
-
Install Shadcn UI CLI: The Shadcn UI CLI tool helps you add components to your project:
npx shadcn-ui@latest init
This command will guide you through a series of prompts to set up your project.
Basic Setup and Configuration
After running the init command, you'll need to configure a few things:
-
Configure Tailwind CSS: Ensure your
tailwind.config.js
file includes the necessary paths:module.exports = { content: [ './pages/**/*.{js,ts,jsx,tsx,mdx}', './components/**/*.{js,ts,jsx,tsx,mdx}', './app/**/*.{js,ts,jsx,tsx,mdx}', ], theme: { extend: {}, }, plugins: [], }
-
Add Tailwind Directives: In your global CSS file (often
globals.css
), add the Tailwind directives:@tailwind base; @tailwind components; @tailwind utilities;
-
Adding Components: Now you're ready to add Shadcn UI components. Use the CLI to add components as needed:
npx shadcn-ui@latest add button
This command will add the button component to your project, creating necessary files and updating your configuration.
-
Using Components: After adding a component, you can import and use it in your React components:
import { Button } from "@/components/ui/button" export default function Home() { return ( <Button>Click me</Button> ) }
-
Customizing Theme: Shadcn UI uses CSS variables for theming. You can customize these in your
globals.css
file::root { --background: 0 0% 100%; --foreground: 222.2 84% 4.9%; /* Add more custom variables here */ }
-
TypeScript Configuration (if using TypeScript): Ensure your
tsconfig.json
includes the necessary paths:{ "compilerOptions": { "baseUrl": ".", "paths": { "@/*": ["./*"] } } }
By following these steps, you'll have Shadcn UI set up in your project and ready to use. The unique approach of Shadcn UI allows you to have full control over the components you add, making it easy to customize and maintain your UI codebase.
Core Components
Shadcn UI offers a rich set of core components that form the building blocks of modern user interfaces. These components are designed to be accessible, customizable, and easy to use. Let's explore some of the key components and dive deeper into a few popular ones.
Overview of Main Components
Shadcn UI provides a wide range of components, including but not limited to:
- Layout: Card, Container, Grid
- Forms: Input, Checkbox, Radio, Select, Textarea
- Navigation: Tabs, Pagination, Breadcrumb
- Feedback: Alert, Progress, Toast
- Overlay: Dialog, Drawer, Popover
- Data Display: Table, Avatar, Badge
- Typography: Heading, Text, List
Each component is crafted with attention to detail, ensuring a balance between functionality and aesthetics.
Detailed Look at Popular Components
Let's examine three widely used components in more detail:
Buttons
Buttons are fundamental to user interaction. Shadcn UI's Button component offers various styles and states:
import { Button } from "@/components/ui/button"
export function ButtonDemo() {
return (
<div>
<Button variant="default">Default</Button>
<Button variant="destructive">Destructive</Button>
<Button variant="outline">Outline</Button>
<Button variant="secondary">Secondary</Button>
<Button variant="ghost">Ghost</Button>
<Button variant="link">Link</Button>
</div>
)
}
The Button component supports different variants, sizes, and can be easily customized to fit your design system.
Forms
Forms are crucial for data input. Shadcn UI provides form components that are both functional and accessible. Here's an example using the Input and Label components:
import { Input } from "@/components/ui/input"
import { Label } from "@/components/ui/label"
export function InputWithLabel() {
return (
<div className="grid w-full max-w-sm items-center gap-1.5">
<Label htmlFor="email">Email</Label>
<Input type="email" id="email" placeholder="Email" />
</div>
)
}
These components work together seamlessly, providing a smooth user experience and adhering to accessibility standards.
Dialog (Modal)
The Dialog component is useful for displaying content that requires user interaction. Here's a basic implementation:
import {
Dialog,
DialogContent,
DialogDescription,
DialogHeader,
DialogTitle,
DialogTrigger,
} from "@/components/ui/dialog"
import { Button } from "@/components/ui/button"
export function DialogDemo() {
return (
<Dialog>
<DialogTrigger asChild>
<Button variant="outline">Open Dialog</Button>
</DialogTrigger>
<DialogContent>
<DialogHeader>
<DialogTitle>Are you sure?</DialogTitle>
<DialogDescription>
This action cannot be undone.
</DialogDescription>
</DialogHeader>
</DialogContent>
</Dialog>
)
}
The Dialog component is highly customizable and includes features like focus management and keyboard navigation out of the box.
Customization Capabilities
One of the strengths of Shadcn UI is the ease with which these components can be customized. You have full access to the component's source code, allowing you to modify styles, behavior, or even core functionality to suit your specific needs.
For instance, you can easily create custom variants for a Button:
import { cva } from "class-variance-authority"
const buttonVariants = cva(
"inline-flex items-center justify-center rounded-md text-sm font-medium transition-colors focus-visible:outline-none focus-visible:ring-2 focus-visible:ring-ring focus-visible:ring-offset-2 disabled:opacity-50 disabled:pointer-events-none ring-offset-background",
{
variants: {
variant: {
default: "bg-primary text-primary-foreground hover:bg-primary/90",
destructive: "bg-destructive text-destructive-foreground hover:bg-destructive/90",
outline: "border border-input hover:bg-accent hover:text-accent-foreground",
secondary: "bg-secondary text-secondary-foreground hover:bg-secondary/80",
ghost: "hover:bg-accent hover:text-accent-foreground",
link: "underline-offset-4 hover:underline text-primary",
custom: "bg-purple-500 text-white hover:bg-purple-700", // Custom variant
},
size: {
default: "h-10 py-2 px-4",
sm: "h-9 px-3 rounded-md",
lg: "h-11 px-8 rounded-md",
},
},
defaultVariants: {
variant: "default",
size: "default",
},
}
)
This level of customization allows you to maintain consistency with your design system while leveraging the robust foundation provided by Shadcn UI.
Customization and Theming
One of the standout features of Shadcn UI is its exceptional flexibility when it comes to customization and theming. This section will explore how you can tailor Shadcn UI components to match your project's unique design requirements.
How to Customize Components
Shadcn UI's "copy and paste" approach gives you direct access to component source code, allowing for deep customization. Here are some key ways to customize components:
-
Modifying Styles: You can easily adjust the styles of any component by editing the Tailwind classes directly in the component file. For example, to change the background color of a button:
<Button className="bg-blue-500 hover:bg-blue-600"> Click me </Button>
-
Creating Variants: Shadcn UI uses the
cva
(class-variance-authority) function to create component variants. You can add or modify variants in the component's definition:const buttonVariants = cva( // ... existing classes ... { variants: { variant: { // ... existing variants ... custom: "bg-purple-500 text-white hover:bg-purple-700", }, }, } )
-
Extending Functionality: Since you have access to the full component code, you can add new props or modify the component's behavior:
interface ButtonProps extends React.ButtonHTMLAttributes<HTMLButtonElement> { isLoading?: boolean; } export function Button({ isLoading, children, ...props }: ButtonProps) { return ( <button {...props}> {isLoading ? <Spinner /> : children} </button> ) }
Theming Capabilities and Best Practices
Shadcn UI leverages CSS variables for theming, making it easy to create and switch between different color schemes. Here's how you can effectively theme your application:
-
Global Theme Variables: Define your theme colors in your global CSS file (e.g.,
globals.css
)::root { --background: 0 0% 100%; --foreground: 222.2 84% 4.9%; --card: 0 0% 100%; --card-foreground: 222.2 84% 4.9%; --popover: 0 0% 100%; --popover-foreground: 222.2 84% 4.9%; --primary: 222.2 47.4% 11.2%; --primary-foreground: 210 40% 98%; --secondary: 210 40% 96.1%; --secondary-foreground: 222.2 47.4% 11.2%; --muted: 210 40% 96.1%; --muted-foreground: 215.4 16.3% 46.9%; --accent: 210 40% 96.1%; --accent-foreground: 222.2 47.4% 11.2%; --destructive: 0 84.2% 60.2%; --destructive-foreground: 210 40% 98%; --border: 214.3 31.8% 91.4%; --input: 214.3 31.8% 91.4%; --ring: 222.2 84% 4.9%; --radius: 0.5rem; }
-
Dark Mode: Implement dark mode by adding a
.dark
class with different color values:.dark { --background: 222.2 84% 4.9%; --foreground: 210 40% 98%; /* ... other dark mode colors ... */ }
-
Custom Color Schemes: Create additional color schemes by defining new CSS classes:
.theme-blue { --primary: 201 96% 32%; --primary-foreground: 0 0% 100%; /* ... other blue theme colors ... */ }
-
Applying Themes: Use JavaScript to toggle theme classes on the
<html>
or<body>
element:document.documentElement.classList.toggle('dark')
-
Component-Specific Theming: For more granular control, you can theme individual components by overriding their CSS variables:
.custom-button { --button-background: 201 96% 32%; --button-foreground: 0 0% 100%; }
Best Practices for Customization and Theming
-
Consistency: Maintain a consistent design language across your application by defining a set of reusable design tokens.
-
Avoid Direct Overwrites: Instead of directly modifying the Shadcn UI component files, create wrapper components that extend the original ones.
-
Use Design Systems: Implement your design system using Shadcn UI as a foundation, creating custom components and variants as needed.
-
Responsive Design: Utilize Tailwind's responsive classes to ensure your customizations work well across different screen sizes.
-
Accessibility: When customizing, ensure that you maintain or improve the accessibility features built into Shadcn UI components.
-
Performance: Be mindful of performance implications when adding custom styles or behaviors. Utilize Tailwind's purge feature to remove unused styles in production.
Performance Considerations
When integrating any UI library into your project, performance is a crucial factor to consider. Shadcn UI is designed with performance in mind, but it's important to understand how to optimize its usage for the best possible user experience. This section will explore the impact of Shadcn UI on application performance and provide optimization techniques.
Impact on Application Performance
Shadcn UI generally has a positive impact on performance due to its unique approach:
-
Minimal Bundle Size: Since you only copy the components you need, there's no unnecessary code bloating your application.
-
CSS-in-JS Free: Shadcn UI uses Tailwind CSS, avoiding the runtime overhead associated with CSS-in-JS libraries.
-
Server-Side Rendering (SSR) Compatible: The components work well with SSR, allowing for faster initial page loads.
-
Reduced Network Requests: With styles included in your bundle, there are fewer network requests for external stylesheets.
However, as with any UI library, improper usage can lead to performance issues. Let's explore some optimization techniques to ensure your application remains fast and responsive.
Optimization Techniques
-
Code Splitting: Use dynamic imports to load Shadcn UI components only when needed:
import dynamic from 'next/dynamic' const DynamicDialog = dynamic(() => import('@/components/ui/dialog')) function MyComponent() { return ( <DynamicDialog> {/* Dialog content */} </DynamicDialog> ) }
-
Tree Shaking: Ensure your bundler is configured to perform tree shaking. This will remove unused Shadcn UI code from your final bundle.
-
Lazy Loading: For complex components or those not immediately visible, consider lazy loading:
import React, { lazy, Suspense } from 'react' const LazyComponent = lazy(() => import('./LazyComponent')) function MyComponent() { return ( <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> ) }
-
Memoization: Use React.memo for components that often receive the same props:
import React from 'react' import { Button } from "@/components/ui/button" const MemoizedButton = React.memo(Button) function MyComponent({ onClick }) { return <MemoizedButton onClick={onClick}>Click Me</MemoizedButton> }
-
Virtual Scrolling: For long lists, consider implementing virtual scrolling to render only visible items:
import { useVirtual } from 'react-virtual' import { ScrollArea } from "@/components/ui/scroll-area" function VirtualList({ items }) { const parentRef = React.useRef() const rowVirtualizer = useVirtual({ size: items.length, parentRef, estimateSize: React.useCallback(() => 35, []), overscan: 5, }) return ( <ScrollArea ref={parentRef} className="h-[200px]"> <div style={{ height: `${rowVirtualizer.totalSize}px`, width: '100%', position: 'relative', }} > {rowVirtualizer.virtualItems.map((virtualRow) => ( <div key={virtualRow.index} style={{ position: 'absolute', top: 0, left: 0, width: '100%', height: `${virtualRow.size}px`, transform: `translateY(${virtualRow.start}px)`, }} > {items[virtualRow.index]} </div> ))} </div> </ScrollArea> ) }
-
Optimizing Images: If you're using images within Shadcn UI components, ensure they're optimized:
import Image from 'next/image' import { Avatar, AvatarImage, AvatarFallback } from "@/components/ui/avatar" function OptimizedAvatar({ src, alt }) { return ( <Avatar> <AvatarImage asChild> <Image src={src} alt={alt} width={40} height={40} /> </AvatarImage> <AvatarFallback>{alt[0]}</AvatarFallback> </Avatar> ) }
-
Debouncing and Throttling: For input components that trigger frequent updates, use debouncing or throttling:
import { useState, useCallback } from 'react' import { debounce } from 'lodash' import { Input } from "@/components/ui/input" function DebouncedInput({ onValueChange }) { const [value, setValue] = useState('') const debouncedChange = useCallback( debounce((value) => onValueChange(value), 300), [onValueChange] ) const handleChange = (e) => { const newValue = e.target.value setValue(newValue) debouncedChange(newValue) } return <Input value={value} onChange={handleChange} /> }
-
Monitoring Performance: Regularly monitor your application's performance using tools like Lighthouse, Chrome DevTools, or specialized React profiling tools to identify and address any performance bottlenecks.
FAQs about Shadcn UI
Q: Is Shadcn UI free to use?
A: Yes, Shadcn UI is open-source and free to use in both personal and commercial projects.
Q: Do I need to know Tailwind CSS to use Shadcn UI?
A: While having knowledge of Tailwind CSS is beneficial, it's not strictly necessary. However, familiarity with Tailwind will help you customize and extend Shadcn UI components more effectively.
Q: Can I use Shadcn UI with other frameworks besides React?
A: Shadcn UI is primarily designed for React. While the styling concepts can be applied to other frameworks, the components themselves are React-specific.
Q: How does Shadcn UI handle accessibility?
A: Shadcn UI components are built with accessibility in mind, including proper ARIA attributes and keyboard navigation. However, it's always important to test and ensure accessibility in your specific implementation.
Q: Can I use Shadcn UI in a TypeScript project?
A: Yes, Shadcn UI fully supports TypeScript and provides type definitions for all components.
Q: How do I update Shadcn UI components?
A: Since Shadcn UI uses a copy-paste approach, updating components involves manually replacing the component files in your project with the latest versions from the Shadcn UI repository.
Q: Does Shadcn UI support server-side rendering (SSR)?
A: Yes, Shadcn UI components are compatible with server-side rendering frameworks like Next.js.
Q: How can I contribute to Shadcn UI?
A: Contributions to Shadcn UI are welcome. You can contribute by submitting issues, proposing new features, or creating pull requests on the project's GitHub repository.
Q: Is there a way to preview Shadcn UI components before adding them to my project?
A: Yes, the Shadcn UI website provides a component showcase where you can view and interact with all available components.
Q: Can I use Shadcn UI with Create React App (CRA)?
A: Yes, you can use Shadcn UI with Create React App. However, you may need to eject or use a tool like CRACO to customize the PostCSS configuration for Tailwind CSS.
Q: How does Shadcn UI handle theming and dark mode?
A: Shadcn UI uses CSS variables for theming, making it easy to implement light and dark modes or custom color schemes. You can toggle themes by changing classes on the root HTML element.
Q: Is Shadcn UI suitable for large-scale applications?
A: Yes, Shadcn UI's modular approach and performance optimizations make it suitable for large-scale applications. However, as with any UI library, proper implementation and optimization practices are crucial for maintaining performance at scale.