What is PM2: A Complete Guide to Node.js Process Management
Introduction
Process management is a critical aspect of running Node.js applications in production environments, and PM2 has emerged as one of the most powerful and widely-adopted solutions in this space. As a robust process manager and production runtime, PM2 has become an essential tool in the modern Node.js ecosystem.
PM2 (Process Manager 2) is an advanced process manager designed specifically for Node.js applications, though it can manage other types of applications as well. It provides a comprehensive suite of features that help developers and operations teams maintain and scale their applications effectively. From basic process management to advanced monitoring capabilities, PM2 addresses many of the challenges faced when running Node.js applications in production.
The importance of process management in Node.js applications cannot be overstated. Node.js applications run in a single thread by default, making them vulnerable to crashes and requiring manual intervention for restarts. Additionally, modern applications need to scale across multiple CPU cores to handle increased load efficiently. This is where PM2 steps in, offering automatic process management, load balancing, and monitoring capabilities that are essential for production-grade applications.
For production environments, PM2 serves as a critical infrastructure component by providing:
- Automatic application restart after crashes
- Load balancing across multiple CPU cores
- Built-in monitoring and logging
- Zero-downtime updates
- Environment management
- Process clustering
Understanding PM2
Definition and Core Features
PM2 is an open-source production process manager for Node.js applications, developed and maintained by the team at Keymetrics (now PM2). It serves as a daemon process manager that helps developers manage and keep their applications online 24/7. At its core, PM2 wraps your Node.js applications in a process management layer that handles many of the complex aspects of running applications in production.
History and Development
First released in 2013, PM2 was created to address the growing needs of Node.js applications in production environments. The project has since grown to become one of the most popular process managers in the Node.js ecosystem, with millions of downloads on npm and a robust community of contributors. The name "PM2" signifies that it's a second-generation process manager, building upon the lessons learned from earlier solutions.
Why Choose PM2?
When compared to other process managers like Forever, Nodemon, or SystemD, PM2 stands out for several reasons:
-
Comprehensive Feature Set: PM2 offers a complete suite of production-ready features, from basic process management to advanced monitoring and deployment tools.
-
Active Development: With regular updates and a strong community backing, PM2 continuously evolves to meet modern application requirements.
-
Easy Integration: PM2 seamlessly integrates with popular development tools and platforms, making it a natural choice for modern development workflows.
-
Production-Ready: It's battle-tested and used by companies of all sizes, from startups to large enterprises.
Key Benefits
For development teams, PM2 offers several significant advantages:
-
Simplified Process Management: Developers can focus on writing code while PM2 handles the complexities of process management.
-
Improved Application Reliability: Automatic restart capabilities and health monitoring ensure applications stay online.
-
Enhanced Performance: Built-in load balancing and clustering capabilities help applications utilize server resources efficiently.
-
Detailed Insights: Comprehensive monitoring and logging features provide visibility into application behavior and performance.
-
Development Workflow Integration: PM2 fits naturally into modern development workflows, supporting various deployment strategies and environments.
Core Features and Capabilities
Process Management
PM2's process management capabilities form the foundation of its functionality. Key process management features include:
-
Application Daemonization: PM2 runs your applications as daemon processes, ensuring they continue running in the background even after you log out of your server.
-
Process Lifecycle Management: Automatically handles process start, stop, restart, and reload operations with simple commands like
pm2 start
,pm2 stop
, andpm2 restart
. -
Automatic Restart: If your application crashes or goes down unexpectedly, PM2 automatically restarts it, ensuring maximum uptime.
Load Balancing
PM2 provides built-in load balancing capabilities that help distribute application load across multiple CPU cores:
-
Cluster Mode: Easily create multiple instances of your application using the Node.js cluster module with a single command:
pm2 start app.js -i max
. -
Smart Load Distribution: PM2 automatically balances requests across all instances of your application, maximizing server resource utilization.
-
Instance Management: Dynamically scale the number of application instances up or down based on load requirements.
Log Management
Comprehensive logging features help developers track application behavior and troubleshoot issues:
-
Centralized Log Management: All application logs are automatically collected and stored in a central location.
-
Log Rotation: Built-in support for log rotation prevents log files from consuming excessive disk space.
-
Real-time Log Monitoring: View logs in real-time using commands like
pm2 logs
with various filtering options.
Monitoring Capabilities
PM2 provides robust monitoring features to help teams maintain optimal application performance:
-
Resource Monitoring: Track CPU usage, memory consumption, and other vital metrics for each process.
-
Performance Metrics: Monitor request rates, response times, and other application-specific metrics.
-
Health Checks: Regular health checks ensure your application is responding correctly.
Zero-downtime Reload
PM2 enables seamless application updates without service interruption:
-
Graceful Reload: Update application code without dropping any user connections using
pm2 reload
. -
Rolling Restarts: When running multiple instances, PM2 performs rolling restarts to maintain service availability.
-
Version Management: Keep track of application versions and easily rollback if issues are detected.
Getting Started with PM2
Installation Process
Installing PM2 is straightforward using Node Package Manager (npm). The global installation ensures PM2 is available system-wide:
npm install pm2 -g
For users preferring Yarn:
yarn global add pm2
Basic Commands and Usage
PM2 provides an intuitive command-line interface for managing applications:
- Starting Applications:
# Basic start
pm2 start app.js
# Start with specific name
pm2 start app.js --name "my-app"
# Start in cluster mode
pm2 start app.js -i 4
- Managing Processes:
# List all processes
pm2 list
# Stop an application
pm2 stop app-name
# Restart an application
pm2 restart app-name
# Delete an application
pm2 delete app-name
Process Configuration
PM2 supports configuration files (ecosystem files) for more complex deployments:
// ecosystem.config.js
module.exports = {
apps: [{
name: "my-app",
script: "./app.js",
instances: 4,
exec_mode: "cluster",
watch: true,
env: {
NODE_ENV: "development",
},
env_production: {
NODE_ENV: "production",
}
}]
}
Start your application using the configuration file:
pm2 start ecosystem.config.js
Environment Management
PM2 makes it easy to manage different environment configurations:
- Environment Variables:
- Set environment-specific variables in the ecosystem file
- Switch between environments using
--env
flag
pm2 start ecosystem.config.js --env production
- Configuration Files:
- Maintain separate configuration files for different environments
- Use environment-specific settings for various deployment scenarios
- Runtime Configuration:
- Modify environment variables on the fly
- Update application configuration without restart
Advanced Features
Cluster Mode
PM2's cluster mode enables sophisticated application scaling capabilities:
// Cluster mode configuration
module.exports = {
apps: [{
script: 'app.js',
instances: 'max', // or a specific number
exec_mode: 'cluster',
instance_var: 'INSTANCE_ID',
wait_ready: true,
listen_timeout: 3000
}]
}
Key cluster features include:
- Automatic load balancing across instances
- Zero-downtime reloads
- Instance failure recovery
- CPU core optimization
Container Integration
PM2 works seamlessly with containerized environments:
- Docker Integration:
- Official Docker images available
- Containerized process management
- Health check endpoints
- Container-aware monitoring
- Kubernetes Support:
- Process management within pods
- Container lifecycle integration
- Resource optimization
Deployment Workflow
PM2 provides robust deployment capabilities:
# Setup deployment configuration
pm2 ecosystem
# Deploy to production
pm2 deploy production
# Rollback if needed
pm2 deploy production revert 1
Deployment features include:
- Multi-server deployment
- Automated deployment scripts
- Version management
- Rollback capabilities
- Pre/post deployment hooks
Monitoring Dashboard
PM2 offers comprehensive monitoring through its built-in web interface:
- Real-time Metrics:
- CPU and memory usage
- Request rates
- Error rates
- Custom metrics
- System Monitoring:
- Server health checks
- Resource utilization
- Network statistics
- Disk usage
Log Rotation
Advanced log management capabilities:
module.exports = {
apps: [{
name: "app",
script: "./app.js",
log_date_format: "YYYY-MM-DD HH:mm Z",
error_file: "./logs/error.log",
out_file: "./logs/out.log",
log_file: "./logs/combined.log",
max_size: "10M",
max_restarts: 10
}]
}
Best Practices
Configuration Recommendations
When using PM2 in production environments, following these configuration best practices is essential:
- Application Configuration:
- Always use ecosystem config files instead of command-line parameters
- Set appropriate memory limits for your applications
- Configure proper error handling and logging
- Use meaningful names for your processes
Example of a well-configured ecosystem file:
module.exports = {
apps: [{
name: 'production-app',
script: './app.js',
instances: 'max',
max_memory_restart: '1G',
max_restarts: 10,
watch: false,
error_file: './logs/error.log',
out_file: './logs/output.log',
time: true
}]
}
Security Considerations
Implementing proper security measures is crucial:
- Process Permissions:
- Run processes with minimum required privileges
- Use separate user accounts for different applications
- Implement proper file permissions
- Environment Variables:
- Never commit sensitive data to version control
- Use environment-specific configuration files
- Implement secure secrets management
- Network Security:
- Configure proper firewall rules
- Implement rate limiting
- Use secure communication protocols
Performance Optimization
Optimize your PM2 setup for maximum performance:
- Resource Management:
- Monitor and adjust memory limits
- Optimize the number of instances based on CPU cores
- Implement proper load balancing strategies
- Application Scaling:
- Use cluster mode effectively
- Implement horizontal scaling when needed
- Monitor and adjust based on load patterns
Common Pitfalls to Avoid
- Process Management:
- Don't ignore application logs
- Avoid running too many instances
- Don't neglect monitoring and alerts
- Configuration Errors:
- Not setting proper environment variables
- Incorrect path configurations
- Missing error handling
- Deployment Issues:
- Not testing deployment scripts
- Ignoring rollback procedures
- Insufficient monitoring during deployments
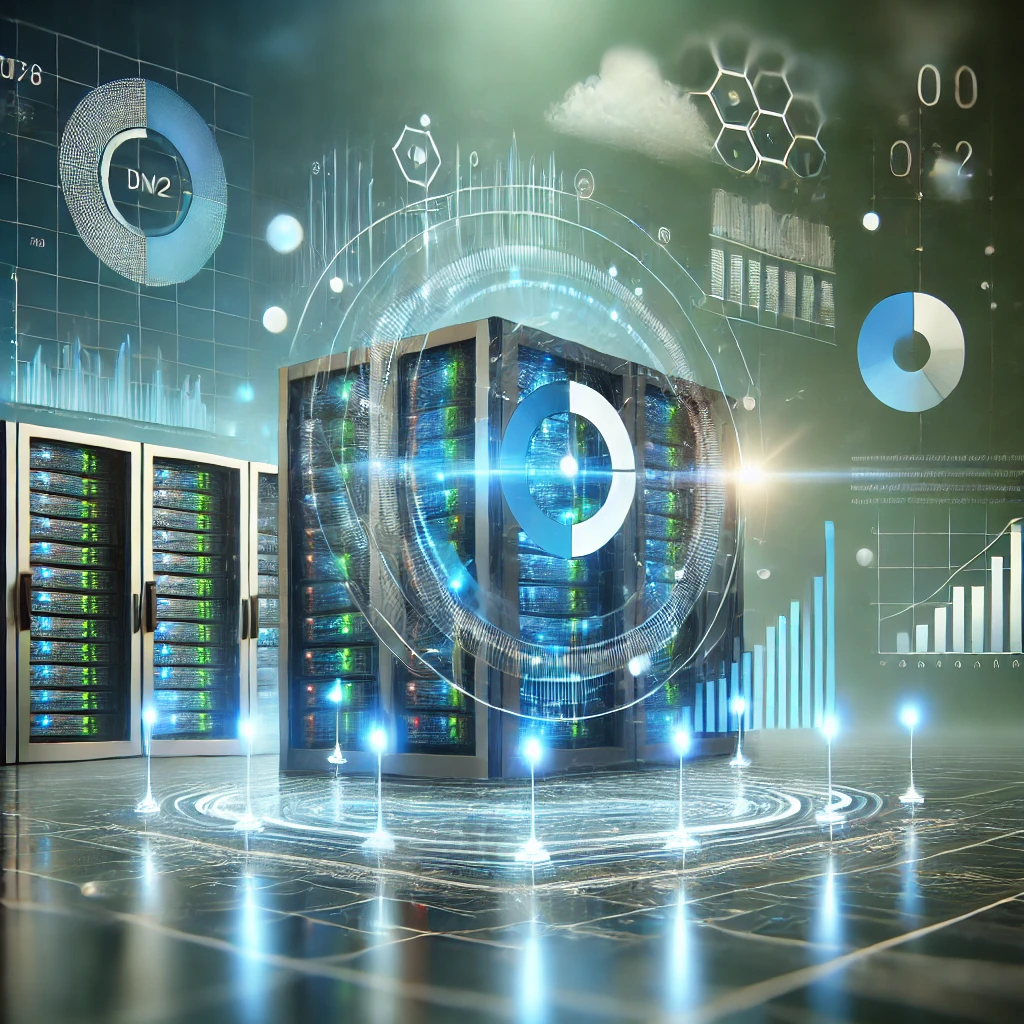
Frequently Asked Questions (FAQ)
1. How do I restart my application when files change?
A: You can use PM2's watch feature:
# Using command line
pm2 start app.js --watch
# Or in ecosystem.config.js
module.exports = {
apps: [{
script: 'app.js',
watch: true,
ignore_watch: ['node_modules', 'logs']
}]
}
2. How can I view logs for a specific application?
A: PM2 provides several logging commands:
# View all logs
pm2 logs
# View logs for specific app
pm2 logs app-name
# View last N lines
pm2 logs --lines 100
3. Why is my application using more memory than expected?
A: This could be due to several reasons:
- Memory leaks in your application
- Too many instances running
- Improper garbage collection
Solution: Use PM2's memory limit feature:
{
"name": "app",
"script": "app.js",
"max_memory_restart": "1G"
}
4. How do I run different configurations in development and production?
A: Use environment-specific configurations:
module.exports = {
apps: [{
script: 'app.js',
env: {
NODE_ENV: 'development',
PORT: 3000
},
env_production: {
NODE_ENV: 'production',
PORT: 80
}
}]
}
5. How can I autostart PM2 after system reboot?
A: Use the startup command:
# Generate startup script
pm2 startup
# Save current process list
pm2 save
6. How do I update PM2 itself?
A: Follow these steps:
# Install latest PM2 version
npm install pm2@latest -g
# Update PM2 in memory
pm2 update
7. Why isn't my Node.js application utilizing all CPU cores?
A: Node.js is single-threaded by default. Use PM2's cluster mode:
# Using command line
pm2 start app.js -i max
# Or in ecosystem.config.js
module.exports = {
apps: [{
script: 'app.js',
instances: 'max',
exec_mode: 'cluster'
}]
}
8. How can I monitor my application's performance?
A: Use PM2's monitoring tools:
# Basic monitoring
pm2 monit
# Web-based dashboard
pm2 plus
# Status overview
pm2 status
9. How do I handle application crashes?
A: PM2 automatically restarts crashed applications, but you can configure specific behaviors:
module.exports = {
apps: [{
script: 'app.js',
max_restarts: 10,
min_uptime: "1m",
restart_delay: 5000
}]
}
10. How can I rotate log files to prevent disk space issues?
A: Configure log rotation in your ecosystem file:
module.exports = {
apps: [{
script: 'app.js',
max_size: "10M",
out_file: "./logs/out.log",
error_file: "./logs/error.log",
merge_logs: true,
time: true
}]
}